How to develop Scalable Programs as a Developer By Gustavo Woltmann
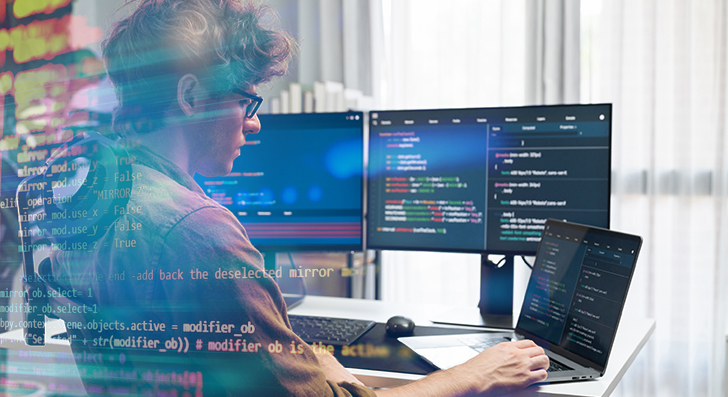
Scalability suggests your application can deal with growth—additional end users, a lot more data, plus more website traffic—with no breaking. For a developer, creating with scalability in your mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your program from the start. Several purposes fail every time they expand rapid since the first style can’t cope with the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Begin by coming up with your architecture for being adaptable. Avoid monolithic codebases in which everything is tightly linked. As a substitute, use modular layout or microservices. These styles break your app into scaled-down, unbiased components. Just about every module or service can scale on its own devoid of impacting The full procedure.
Also, take into consideration your databases from working day one. Will it require to manage 1,000,000 people or perhaps 100? Select the suitable style—relational or NoSQL—depending on how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them nonetheless.
Another essential place is to stay away from hardcoding assumptions. Don’t generate code that only works under present situations. Think of what would occur In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that guidance scaling, like concept queues or party-pushed devices. These enable your application take care of far more requests with no acquiring overloaded.
Once you Construct with scalability in your mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A nicely-planned procedure is simpler to take care of, adapt, and increase. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the right databases can be a critical Section of developing scalable purposes. Not all databases are designed the identical, and using the Erroneous one can gradual you down as well as trigger failures as your app grows.
Get started by knowledge your info. Can it be hugely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are solid with relationships, transactions, and regularity. They also guidance scaling methods like browse replicas, indexing, and partitioning to deal with extra site visitors and details.
In the event your info is more versatile—like person activity logs, merchandise catalogs, or documents—take into account a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing massive volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your browse and create designs. Are you presently carrying out numerous reads with much less writes? Use caching and browse replicas. Are you presently handling a large produce load? Look into databases that may take care of superior write throughput, and even celebration-primarily based info storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel forward. You might not will need Highly developed scaling attributes now, but selecting a databases that supports them suggests you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And always monitor database performance as you grow.
In short, the right database depends on your application’s composition, velocity needs, and how you expect it to mature. Choose time to select correctly—it’ll preserve plenty of problems later.
Improve Code and Queries
Speedy code is key to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated Alternative if an easy just one operates. Keep your features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—sites the place your code requires much too extensive to run or takes advantage of excessive memory.
Subsequent, check out your database queries. These generally slow points down over the code alone. Make certain Each individual question only asks for the data you really have to have. Stay away from Find *, which fetches almost everything, and instead pick unique fields. Use indexes to speed up lookups. And prevent doing too many joins, Primarily across large tables.
Should you see exactly the same knowledge being requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached therefore you don’t have to repeat pricey operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers plus more traffic. If everything goes by means of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching come in. These two applications assistance keep the app quickly, stable, and scalable.
Load balancing spreads incoming visitors throughout numerous servers. As opposed to a single server performing all of the work, the load balancer routes buyers to unique servers based upon availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing data quickly so it may be reused quickly. When people request the same facts once again—like a product site or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t transform frequently. And always be sure your cache is current when info does transform.
In short, load balancing and caching are basic but powerful resources. Jointly, they assist your app take care of extra consumers, keep speedy, and Recuperate from complications. If you plan to expand, you require both.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application improve conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, decrease setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t must get components or guess long run ability. When website traffic improves, you could increase far more methods with just a couple clicks or routinely working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to developing your app instead of running infrastructure.
Containers are A further key tool. A container offers your app and every little thing it must operate—code, libraries, configurations—into one particular unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Whenever your app works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual parts of your application into solutions. You could update or scale areas independently, and that is great for effectiveness and reliability.
To put it briefly, making use of cloud and container applications signifies you can scale rapidly, deploy easily, and Get well quickly when troubles happen. In order for you your app to expand without the need of limitations, start out utilizing these instruments early. They preserve time, cut down threat, and assist you stay focused on making, not fixing.
Check Anything
If you don’t check your software, you received’t know when issues go Mistaken. Checking helps you see how your app is doing, location issues early, and make greater conclusions as your application grows. It’s a important Portion of making scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—observe your application too. Keep an eye on just how long it requires for end users to load web pages, how frequently glitches transpire, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified straight away. This allows you deal with challenges speedy, generally ahead of consumers even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you could roll it back again just before it causes serious hurt.
As your app grows, targeted visitors and knowledge here boost. Without checking, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps require a robust foundation. By planning diligently, optimizing properly, and utilizing the right equipment, you can Create applications that develop efficiently without the need of breaking under pressure. Start off small, Feel major, and build wise.